Lets create our first Swift Package !
iOS Developer level : Intermediate
Configuration when this article was written
Xcode : Version 11.5
MacOS : 10.15.5 (Catalina)
A working example
Final code can be found in the Resources section below.
What are Swift Packages ?
Swift packages are reusable components of Swift, Objective-C, Objective-C++, C, or C++ code that developers can use in their projects. They bundle source files, binaries, and resources in a way that’s easy to use in your app’s project.
Enough of introduction , let’s be technical…
Xcode -> New Project -> Single View App -> MySwiftPackageCreator
public class DateFormat {
public static func formatDate(dateToFormat date : Date) -> String {
let dateFormat = DateFormatter()
dateFormat.dateFormat = "YYYY-MM-dd"
return dateFormat.string(from: date)
}
}
The class and method is marked public because we will have this file bundled in swift package and the other modules later accessing our swift package will have access to this.
Also formatDate is simple method to format a date passed into "YYYY-MM-dd" format.
Now in change the viewDidLoad() in ViewController.swift to look like below
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
print("Formatted date is \(DateFormat.formatDate(dateToFormat: Date()))")
}
Now build the Xcode project . You will see
Step 2 : Create Swift Pacakage
Now Add new Swift Package to the same project. To do this follow the screenshots below.
.library(
name: "TTDateFormatter",
targets: ["TTDateFormatter"]),
So the name in .library is place where all the package files are present. Do not change this name.
Now delete the file TTDateFormatter.swift in TTDateFormatter folder.
Drag the file DateFormat.swift into TTDateFormatter folder. Also copy to folder
Now build the project yow will see the error "Use of unresolved identifier 'DateFormat'" in ViewController.swift
This is because 'DateFormat' is now moved to Swift Package.
Add import TTDateFormatter, in ViewController.swift.
Now the error should go away. if the error persists change the target to TTDateFormatter and rebuild the xcode project.
README.md file.
git tag 1.0.0
git push --tags
Now we have our TTDateFormatter package on Github.
Xcode -> New Project -> Single View App -> MyPackageTestFromRemote
Now go to project genral settings screen and click on add framework button
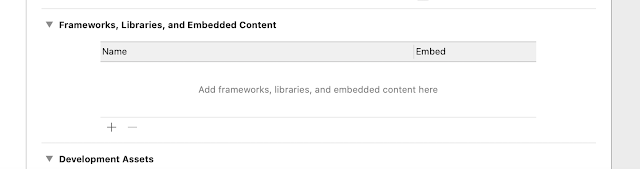
In the popup showing place to enter Package Repository Url add the url where SwiftPackage is hosted for me it is https://github.com/TeaTalkInternal/TTDateFormatter.git
Now the package is added .
Now open ViewController.swift change the code to look like below.
import UIKit
import TTDateFormatter
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
print("Formatted date is \(DateFormat.formatDate(dateToFormat: Date()))")
}
}
Run and see the result. You will see the Formatted date is 2020-08-15 printed the console.
Yes we successfully created swift package and used it too.
We stop here ...
Resources:
https://github.com/TeaTalkInternal/TTDateFormatter
My Reference :
https://www.youtube.com/watch?v=xu9oeCAS8aA
great! stuff here.
ReplyDeleteThanks :)
Delete